30+ Python Tips and Tricks for Beginners
Introduction
Unlocking your coding potential requires investigating the syntax and feature set of Python. You may optimize your code for performance and elegance by learning basic concepts like list comprehensions, generating expressions, and unpacking. Explore a vast array of carefully selected 30+ Python tips and tricks that are sure to improve your programming skills and enable you to write clear, efficient, and Pythonic code that appeals to both novice and experienced developers.
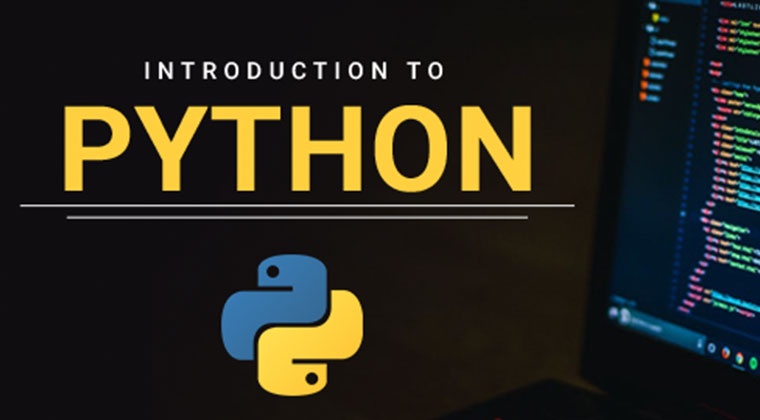
You can also enroll in our free python course today!
30+ Python Tips and Tricks
1. List Comprehensions
List comprehensions in Python provide a streamlined approach to creating lists from pre-existing iterables. One or more for or if clauses, an expression, and then another expression make up their composition. They offer an alternative to traditional looping algorithms for list-building that is both more concise and comprehensible.
Use list comprehensions for concise creation of lists.
squares = [x**2 for x in range(10)]
print(squares)
# Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
2. Dictionary Comprehensions
Python dictionary comprehensions let you build dictionaries in a clear and expressive way. They generate dictionaries instead of lists, although they use a syntax similar to that of list comprehensions. Within curly braces {}, you specify key-value pairs where a colon (:) separates the key and value.
squares_dict = {x: x**2 for x in range(10)}
print(squares_dict)
# Output: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
3. Generator Expressions
Generator expressions of python work similarly to list comprehensions. But they yield a generator object as opposed to a list. They provide a memory-efficient method of creating data instantly, which is very helpful for big datasets. Generator expressions replace square brackets [] with parentheses () in Python.
squares_gen = (x**2 for x in range(10))
for num in squares_gen:
print(num)
# Output: 0 1 4 9 16 25 36 49 64 81
4. Unpacking
Unpacking in python enables you to take individual elements out of iterables, such lists or tuples, and put them into variables. When you have data sets and wish to access their individual components independently, you frequently use it.
a, b, c = [1, 2, 3]
print(a, b, c)
# Output: 1 2 3
5. Multiple Assignments in One Line
In Python, you can give values to numerous variables in a single statement by using multiple assignments in one line. When you wish to assign numerous values at once, using different or the same data types, this is quite helpful.
a, b = 1, 2
print(a, b)
# Output: 1 2
6. Enumerate
An enumerate object, which comprises pairs of indices and values from an iterable. The enumerate() function in Python returns it. It’s especially helpful when you have to iterate over an iterable while maintaining track of each element’s index or position.
for index, value in enumerate(squares):
print(index, value)
# Output: (0, 0) (1, 1) (2, 4) (3, 9) (4, 16) (5, 25) (6, 36) (7, 49) (8, 64) (9, 81)
7. Zip
Zip function of python is a built-in function. It takes as input two or more iterables and outputs an iterator that builds tuples in the output tuple with the i-th element from each of the input iterables.
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
for a, b in zip(list1, list2):
print(a, b)
# Output: 1 a 2 b 3 c
8. zip(*iterables) Unpacking Trick
Python’s zip(*iterables) unpacking function enables the conversion of data from rows to columns or vice versa. It takes many iterables as input and outputs an iterator that takes each input iterable that makes up the i-th tuple and uses it to create tuples with the i-th element.
matrix = [[1, 2], [3, 4], [5, 6]]
transposed = zip(*matrix)
print(list(transposed))
# Output: [(1, 3, 5), (2, 4, 6)]
9. collections.Counter
The built-in Python class collections.Counter is utilized to count the occurrences of elements in a collection, usually in iterables such as lists or strings. It provides a convenient way to perform frequency counting in a Pythonic and efficient manner.
from collections import Counter
counts = Counter([1, 2, 1, 3, 1, 2, 4])
print(counts)
# Output: Counter({1: 3, 2: 2, 3: 1, 4: 1})
10. collections.defaultdict
collections.defaultdict is a Python subclass that provides a default value for each key in a dictionary, eliminating the need to check if a key exists, especially useful for nested dictionaries or missing keys.
from collections import defaultdict
d = defaultdict(list)
d['key'].append('value')
print(d['key'])
# Output: ['value']
11. collections.namedtuple
collections.namedtuple is a factory function for creating tuple subclasses with named fields. Named tuples are a convenient method for defining simple classes without explicitly writing a full definition. Named tuples are immutable, but you can access their fields using dot notation, making them more readable and self-documenting.
from collections import namedtuple
Point = namedtuple('Point', ['x', 'y'])
p = Point(1, y=2)
print(p)
# Output: Point(x=1, y=2)
12. any() and all()
any()
: This function returns True if at least one element in the iterable evaluates to True. If the iterable is empty, it returns False. It’s useful when you want to check if any of the elements in the iterable satisfy a condition.all()
: This function returns True if all elements in the iterable evaluate to True. If the iterable is empty, it returns True. It’s useful when you want to check if all elements in the iterable satisfy a condition.
print(any([True, False, True]))
# Output: True
print(all([True, False, True]))
# Output: False
13. sorted()
The built-in Python function sorted() is used to return a new sorted list after sorting iterables such as lists, tuples, and strings. We can also pass optional arguments to customize the sorting behavior.
sorted_list = sorted([3, 1, 4, 1, 5, 9, 2])
print(sorted_list)
# Output: [1, 1, 2, 3, 4, 5, 9]
14. filter()
A built-in Python function called filter() can be used to apply a given function to filter elements from an iterable. When the function returns True, it returns an iterator that yields the elements of the iterable.
filtered_list = list(filter(lambda x: x > 5, [3, 8, 2, 7, 1]))
print(filtered_list)
# Output: [8, 7]
15. map()
You can obtain an iterator yielding the results of each item in an iterable by using the built-in Python function map().
doubled_list = list(map(lambda x: x * 2, [1, 2, 3, 4]))
print(doubled_list)
# Output: [2, 4, 6, 8]
16. functools.reduce()
You can use the built-in Python function functools.reduce() to reduce an iterable to a single value by applying a function with two arguments cumulatively on its members from left to right.
from functools import reduce
product = reduce((lambda x, y: x * y), [1, 2, 3, 4])
print(product)
# Output: 24
17. *args and **kwargs
*args
: It is used to pass a variable number of positional arguments to a function, allowing it to accept any number of arguments and collect them into a tuple when used as a parameter in a function definition.**kwargs
: It is a notation used to pass a variable number of keyword arguments to a function, allowing it to accept any number of arguments and collect them into a dictionary when used as a parameter in a function definition.
def my_func(*args, **kwargs):
print(args, kwargs)
my_func(1, 2, a=3, b=4)
# Output: (1, 2) {'a': 3, 'b': 4}
18. .__doc__
The docstring (documentation string) of a class, function, module, or method can be accessed using the unique attribute.doc. It offers a means of describing the functionality and documentation of Python programming.
def my_func():
"""This is a docstring."""
pass
print(my_func.__doc__)
# Output: This is a docstring.
19. dir()
A robust built-in Python function called dir() retrieves the names of the attributes of an object or a list of names in the current local scope. It’s frequently employed for debugging, investigation, and introspection.
print(dir([]))
# Output: ['__add__', '__class__', '__contains__', ...]
20. getattr()
A built-in Python function called getattr() returns the value of an object’s designated attribute. In addition, it offers a default value in the event that the attribute is absent.
class MyClass:
attribute = 42
print(getattr(MyClass, 'attribute'))
# Output: 42
21. setattr()
To set an object’s named attribute value, use the built-in Python function setattr().
It enables you to dynamically set the value of an attribute on an object, even if the attribute hasn’t been declared yet.
class MyClass:
pass
obj = MyClass()
setattr(obj, 'attribute', 42)
print(obj.attribute)
# Output: 42
22. hasattr()
The hasattr() built-in Python function can be used to determine whether an object has a specific attribute or not. It returns True if the object has the attribute and False otherwise.
class MyClass:
attribute = 42
print(hasattr(MyClass, 'attribute'))
# Output: True
23. __str__
and __repr__
In Python, developers use two specific methods called str and repr to define string representations of objects. These methods are immediately called when you use functions like print() or str(), or when an object is inspected in the interpreter.
- __str__(self): The str() function calls this method to return a string representation of the object for end-users. It should return a human-readable representation of the object.
- __repr__(self): The repr() function returns an unambiguous string representation of an object, typically used for debugging and logging. The returned string should be valid Python code that can recreate the object if passed to eval().
class MyClass:
def __str__(self):
return 'MyClass'
def __repr__(self):
return 'MyClass()'
obj = MyClass()
print(str(obj), repr(obj))
# Output: MyClass MyClass()
24. *
and **
Operators
In Python, the * (asterisk) and ** (double asterisk) operators are used for unpacking iterables and dictionaries, respectively, in function calls, list literals, tuple literals, and dictionary literals. They are powerful tools for working with variable-length argument lists and dictionary unpacking.
def my_func(a, b, c):
print(a, b, c)
my_list = [1, 2, 3]
my_dict = {'a': 1, 'b': 2, 'c': 3}
my_func(*my_list)
my_func(**my_dict)
# Output: 1 2 3
# 1 2 3
25. pass Statement
The pass statement in Python is a null operation that does nothing when executed, serving as a placeholder for syntactically required statements without any action needed, often used for unimplemented code or empty code blocks.
def my_func():
pass
my_func()
26. with Statement
The with statement in Python streamlines exception handling and resource management by ensuring proper initialization and cleanup, making it useful for managing files, network connections, and other resources to prevent errors.
with open('file.txt', 'r') as f:
data = f.read()
27. __init__.py
The init.py file is a special Python file that is used to define a package. It can be empty or contain initialization code for the package.
# In a directory called 'my_package'
# __init__.py can be empty or contain initialization code
28. @staticmethod
In Python, developers define static methods inside a class using the @staticmethod decorator.
These methods, called static methods, belong to the class and can be directly called on the class itself, without requiring access to instance variables or methods.
class MyClass:
@staticmethod
def my_method():
print('Static method called')
MyClass.my_method()
# Output: Static method called
29. @classmethod
In Python, developers define class methods inside a class using the @classmethod decorator. These methods, known as class methods, are connected to the class itself rather than individual class instances. They can be called on both the class and its instances, and they have access to the class itself through the cls parameter.
class MyClass:
@classmethod
def my_method(cls):
print('Class method called')
MyClass.my_method()
# Output: Class method called
30. is and is not
Python comparison operators is and is not are used to verify the identity of an object. Rather of determining if two objects have the same value, they determine if they correspond to the same memory address.
- is: If two variables belong to the same memory item, this operator returns True; otherwise, it returns False.
- is not: If two variables do not correspond to the same object in memory, this operator returns True; if they do, it returns False.
x = None
print(x is None)
# Output: True
31. del Statement
In Python, developers use the del statement to delete objects. It can remove elements from lists, slices from lists or arrays, delete entire variables, and perform other actions.
my_list = [1, 2, 3]
del my_list[1]
print(my_list)
# Output: [1, 3]
32. lambda Functions
Lambda functions in Python are small, anonymous functions defined using the lambda
keyword. They are typically used for short, one-time operations where defining a named function is unnecessary. Lambda functions can take any number of arguments, but can only have a single expression.
square = lambda x: x**2
print(square(5))
# Output: 25
33. try, except, else, finally
In Python, the try
, except
, else
, and finally
blocks are used for handling exceptions and executing cleanup code in case of exceptions or regardless of whether exceptions occur.
Here’s an explanation of each block in active voice:
try
block: In Python, developers use the try block to catch exceptions during execution, enclosing the code that might raise them and attempting to execute it.except
block: In Python, developers utilize the except block to handle exceptions within the try block, executing the code inside the except block if it matches the exception type.else
block: Theelse
block is executed if the code inside thetry
block executes successfully without raising any exceptions. Developers typically use this block to execute code only if no exceptions occur.finally
block: The finally block is a crucial block in programming that executes cleanup operations, such as closing files or releasing resources, regardless of exceptions.
try:
x = 1 / 0
except ZeroDivisionError:
print('Error: division by zero')
else:
print('No exceptions raised')
finally:
print('This always runs')
# Output: Error: division by zero
# This always runs
Conclusion
The vast array of methods and capabilities that the Python programming language offers is far more than just the handy hints and suggestions. You may improve as a Python developer and learn more about the language’s possibilities by applying these pointers to your code. Both novice and seasoned developers can benefit from these pointers, which will help you advance your Python knowledge and programming skills. These 30+ python tips and tricks will guide you and act as a catalyst in your coding journey.